Build a Successful Single-Page Application Using React.js
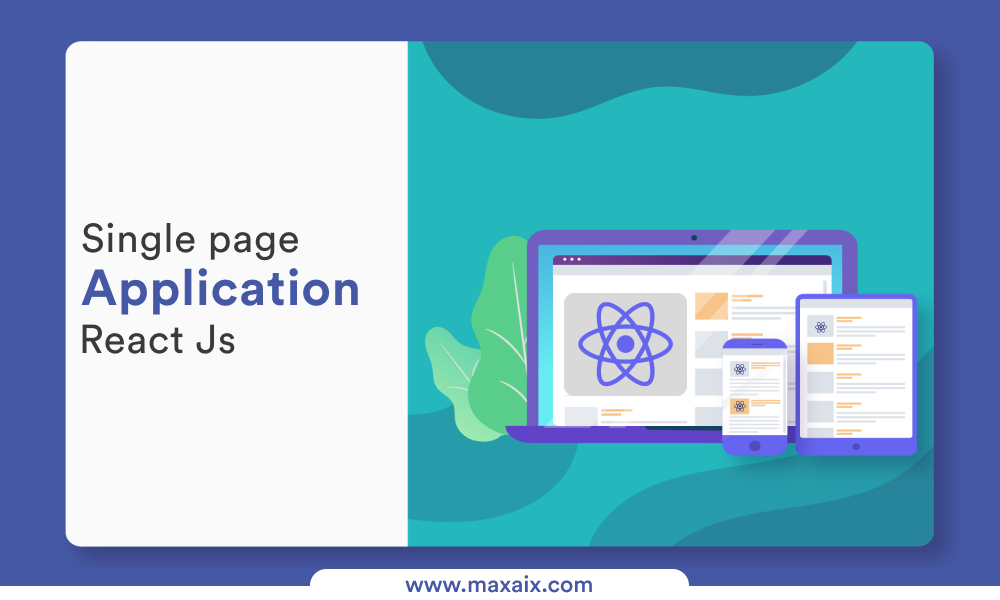
What Is a Single-Page Application?
A Single-Page Application (SPA) is a web application or website that interacts with the user by dynamically rewriting the current page rather than loading entire new pages from the server. SPAs provide a seamless and responsive user experience by loading only the necessary content, minimizing page reloads.
What is the difference between a single-page application and a multi-page application?
Single-Page Application (SPA):
- Dynamic Loading: Loads content dynamically as the user interacts with the app.
- Faster Navigation: Eliminates the need to reload entire pages, leading to faster navigation.
- Enhanced User Experience: Provides a more seamless and interactive user experience.
Multi-Page Application (MPA):
- Traditional Approach: Navigates by loading entirely new pages from the server.
- Slower Response: May experience slower response times due to full page reloads.
- More Server Requests: Involves more requests to the server for each page.
Why should we use an SPA application?
a. The Evolution of Web Applications
The traditional multi-page model of web applications involves loading a new HTML page for each user interaction, resulting in a full page reload. SPAs, on the other hand, offer a more seamless and dynamic experience by loading only the necessary content, eliminating the need for constant page refreshes.
b. Enhanced User Experience
SPAs provide a fluid and interactive user experience akin to that of desktop applications. With content dynamically loaded and updated, users can navigate through the application with minimal interruptions, leading to a smoother and more engaging interface.
c. Faster Response Times
By leveraging client-side rendering and AJAX (Asynchronous JavaScript and XML) requests, SPAs significantly reduce the time it takes for users to interact with the application. This results in faster response times and a more responsive feel, contributing to a positive user perception.
d. Improved Performance on Mobile Devices
As mobile usage continues to rise, optimizing web applications for mobile devices becomes crucial. SPAs excel in this aspect by delivering a more efficient and mobile-friendly experience, ensuring a consistent performance across various devices.
e. Streamlined Development Workflow
The development of SPAs often follows a more streamlined workflow. The separation of the frontend and backend aspects allows for parallel development, enabling frontend developers to work independently without waiting for backend functionalities to be fully implemented.
On which applications are SPAs used?
SPAs are suitable for various applications, including:
- Social Media Platforms: Enhances user interaction and real-time updates.
- E-commerce Websites: Facilitates seamless product browsing and checkout processes.
- Streaming Platforms: Provides uninterrupted content delivery.
- Productivity Tools: Ensures a responsive and efficient user interface.
What are the advantages of a SPA (single-page application)?
1. Seamless Navigation
One of the primary advantages of SPAs is their seamless navigation. Users can explore different sections of the application without experiencing the traditional delays associated with full-page reloads. This seamless flow contributes to a more intuitive and enjoyable user journey.
2. Dynamic Content Loading
SPAs excel in delivering dynamic content loading. Instead of loading entire pages, only the necessary data is fetched and rendered, resulting in faster loading times. This approach enhances the overall responsiveness of the application.
3. Efficient Resource Utilization
SPAs optimize resource utilization by loading essential assets upfront and fetching additional resources as needed. This approach minimizes unnecessary data transfer, reducing the load on servers and improving overall application efficiency.
4. Smooth Transition Between Views
With SPAs, transitioning between views is fluid and instantaneous. User interactions, such as clicking on navigation links, result in swift transitions without the jarring interruptions caused by traditional page reloads. This contributes to a polished and professional user experience.
5. Enhanced Caching Strategies
SPAs can implement effective caching strategies, reducing the need to fetch redundant data from the server. Caching mechanisms enhance performance and decrease load times, especially for users revisiting the application or navigating between different sections.
6. Facilitates Modern Design Patterns
SPAs align well with modern design patterns, enabling the implementation of advanced UI/UX features. Features like real-time notifications, collaborative editing, and immersive multimedia experiences are seamlessly integrated into SPAs.
7. Scalability and Maintainability
The modular structure of SPAs, often facilitated by frameworks like React.js, contributes to scalability and maintainability. Developers can efficiently manage and update individual components, making it easier to scale the application and introduce new features.
When should you avoid using single-page applications?
Considerations to Avoid SPA:
- SEO Requirements: If extensive search engine optimization is crucial, traditional multi-page applications might be more suitable.
- Complex State Management: For applications with complex state management that benefits from server-side rendering.
- Limited Interactivity: If the application doesn’t require frequent user interactions and updates.
React’s Contribution to the Making of a Single-Page Application
React, a JavaScript library for building user interfaces, plays a crucial role in developing SPAs. Its key contributions include:
Component-Based Architecture: Enables building reusable UI components for consistent design.
Virtual DOM: Optimizes rendering by updating only changed parts of the DOM.
Efficient State Management: Utilizes a unidirectional data flow for efficient state management.
React Router: Facilitates easy navigation and routing in SPAs.
Life Cycle of SPA
The life cycle of an SPA involves the following phases:
- Initialization: Sets up the application and initializes components.
- Routing: Handles navigation and routing through the application.
- Rendering: Renders components based on user interactions.
- State Management: Manages the state of the application.
- Cleanup: Handles cleanup tasks and resource management.
Setting Up the Development Environment
Before building an SPA using React, set up the development environment by installing Node.js, npm, and a code editor. Create a new React project using the create-react-app command for a quick start.
Building the User Interface with React Components
Design the user interface by creating reusable React components. Follow best practices for component structure, styling, and responsiveness to ensure a cohesive and visually appealing UI.
Building a Single-Page Application with React Using React Router
a. Basic Information
React Router is a standard library for routing in React applications. It enables navigation between components without triggering a full page reload.
b. Source Code
Use the following sample code to implement basic routing in a React SPA:
import { BrowserRouter as Router, Route, Switch } from ‘react-router-dom’;
function App() {
return (
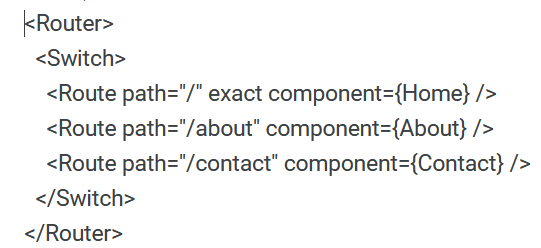
);
}
c. React Part
Define components for Home, About, and Contact pages and ensure proper rendering based on the route.
Routing in Single-Page Applications
Routing in SPAs is handled by client-side routers like React Router. This allows for dynamic loading of content based on user navigation without the need for server requests.
Managing State with React Hooks
React Hooks, introduced in React 16.8, simplify state management in functional components. Use hooks like useState and useEffect to manage and update component state efficiently.
State Management in React SPA
Effective state management is crucial for SPAs. Utilize state management libraries like Redux for centralized and predictable state management, especially in larger applications.
Security Considerations in React SPA
To enhance the security of React SPAs, consider implementing measures such as:
Authentication: Implement secure user authentication.
Authorization: Control access to specific features based on user roles.
HTTPS: Ensure secure data transmission using HTTPS.
Input Validation: Validate user inputs to prevent security vulnerabilities.
Performance Optimization in React SPA
Optimizing performance is essential for a seamless user experience. Implement the following strategies:
- Code Splitting: Split code into smaller chunks for efficient loading.
- Lazy Loading: Load components only when needed.
- Minification: Minify JavaScript and CSS files.
- Image Optimization: Compress and optimize images for faster loading.
Testing Single-Page Applications with React
Ensure the reliability and functionality of your React SPA through thorough testing. Utilize testing libraries like Jest and React Testing Library for unit and integration testing.
Examples of Creating a Single-Page Application Using ReactJS
Explore real-world examples of successful SPAs built with React, such as Facebook, Instagram, and Airbnb. Analyze their architecture, design patterns, and user experience to gain insights into building effective SPAs.
Comparisons of Single-Page Applications with Traditional Web Applications
Compare the pros and cons of SPAs with traditional web applications to make informed decisions based on project requirements.
Single-Page Applications (SPAs):
Pros: Faster navigation, enhanced user experience, efficient resource utilization.
Cons: Initial load time, SEO challenges, potential for increased client-side complexity.
Traditional Web Applications:
Pros: SEO-friendly, straightforward server-side rendering, simpler initial setup.
Cons: Slower navigation, full page reloads, increased server load.
Expert Advice for Building Single-Page Applications Using ReactJS
Follow these expert tips for successful SPA development with React:
- Optimize Performance: Prioritize performance optimization strategies.
- Responsive Design: Ensure a responsive design for various devices.
- Secure Authentication: Implement secure user authentication mechanisms.
- Regular Updates: Keep dependencies and libraries up to date for security and compatibility.
- User Feedback: Gather and incorporate user feedback for continuous improvement.
How Does Maxaix Help You Develop Single-Page Applications Using ReactJS?
Maxaix offers comprehensive support for SPA development using ReactJS. Our services include:
- Consultation: Expert guidance on SPA architecture and development strategies.
- Development: Skilled developers for efficient and scalable React-based SPAs.
- Testing: Rigorous testing procedures for reliable and bug-free applications.
- Maintenance: Ongoing support and maintenance to ensure optimal performance.
Frequently Asked Questions
Q1: What are the key considerations for designing a responsive UI in a React SPA?
A1: Prioritize flexible grid systems, media queries, and responsive design patterns to ensure a seamless user experience across devices.
Q2: Can I integrate third-party libraries into my React SPA?
A2: Yes, React allows the integration of various third-party libraries to enhance functionality and features.
Q3: How can I ensure data security in my React SPA?
A3: Implement secure communication protocols (HTTPS), validate user inputs, and use secure authentication methods to ensure data security.
Q4: What are the best practices for optimizing the performance of a React SPA?
A4: Practice code splitting, lazy loading, and minification. Optimize images and prioritize efficient resource loading.
Q5: Is server-side rendering possible in React SPAs?
A5: While React SPAs primarily render on the client side, frameworks like Next.js enable server-side rendering for improved SEO and initial load performance.
Q6: Can Maxaix assist with ongoing maintenance and updates for my React SPA?
A6: Yes, Maxaix provides ongoing support and maintenance services to ensure your React SPA stays up to date and performs optimally.
Q7: How do I handle SEO challenges in React SPAs?
A7: Implement server-side rendering or use frameworks like Next.js to address SEO challenges in React SPAs.
Q8: What are the key considerations for securing user authentication in React SPAs?
A8: Use secure authentication protocols (OAuth, JWT), encrypt sensitive data, and implement measures to prevent common security threats.
Q9: How can I enhance the accessibility of my React SPA?
A9: Follow accessibility best practices, including semantic HTML, proper use of ARIA roles, and testing with screen readers to ensure an inclusive user experience.
Conclusion
Building a successful single-page application using React.js requires a thorough understanding of the technology, best practices, and user experience considerations. By following the outlined steps, leveraging React’s capabilities, and seeking assistance from experienced development partners like Maxaix, you can create a highly functional and engaging SPA that meets the needs of modern web applications. Stay updated on industry trends, continuously optimize your application, and prioritize user satisfaction for sustained success in the dynamic world of web development.